Python Testing with PyTest
I recently read the PyTest book. It is quite good. If you are looking to learn Python, I recommend checking out PyTest. Unit Tests are a great tool to help learning a new language.
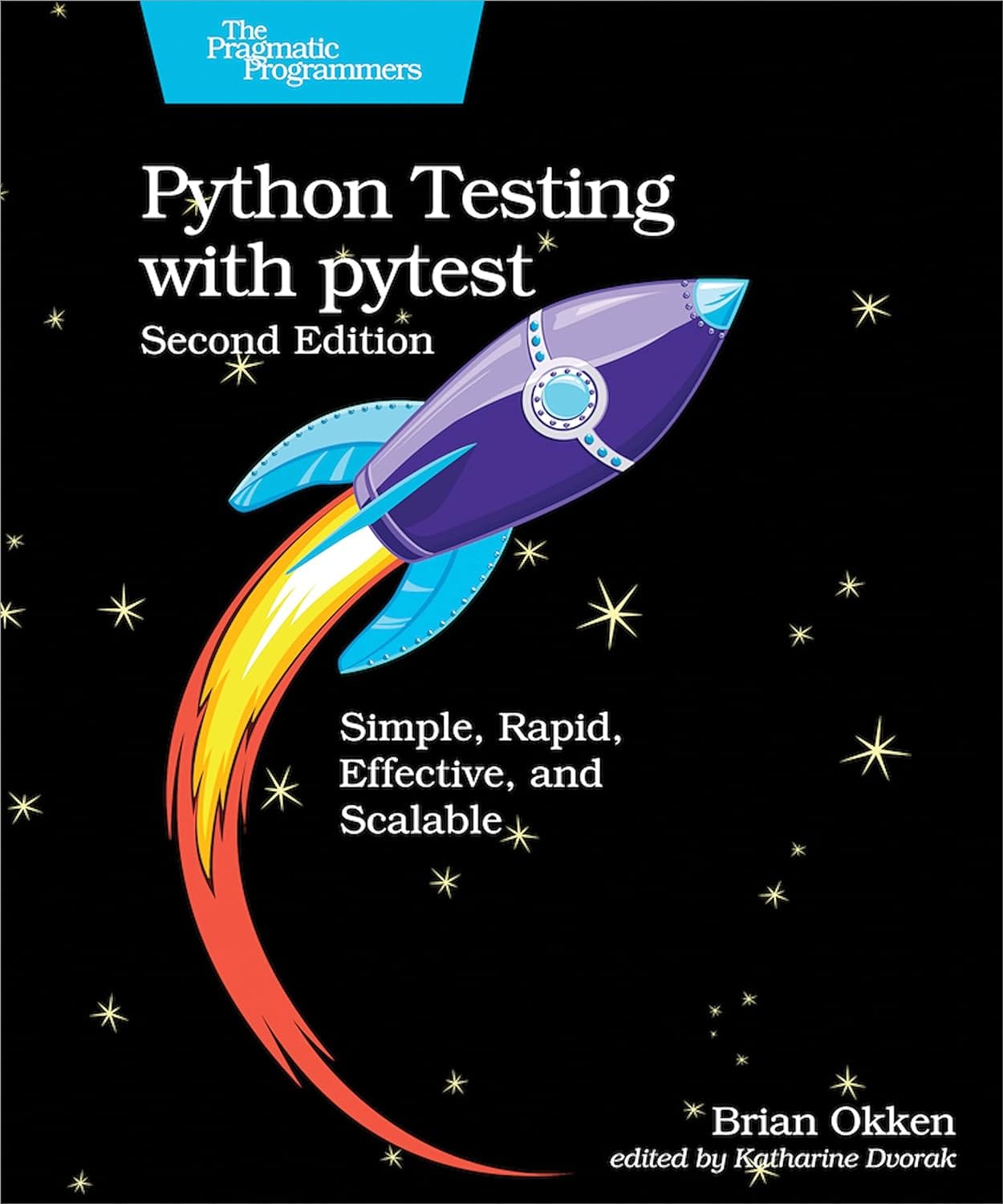
As many of you know, I've been working on my Python skills for quite a while. One of the things I've found really useful for learning a new language is writing unit tests. It helps me avoid a lot of the gotchas in language. I can make sure that the code I write does exactly what I think it does.
UnitTest versus Pytest
In Python there are several unit test frameworks. The 2 most popular are UnitTest (which ships with Python) and PyTest. LabVIEW is similar we have VITester (or its modern replacement LUnit) and Caraya. LUnit and UnitTest are the more traditional XUnit style testers which rely heavily on a class hierarchy while Caraya and PyTest are much more free form and give you much more flexibility. Interestingly enough in LabVIEW I prefer LUnit and in Python I prefer PyTest. In both cases, I think it is just what I learned first in each language.
The Book
I had already played around with PyTest before I picked up this book and I still learned a lot. The book has 3 sections. The first section talks about some very useful features like parameterization, fixtures and running subsets of tests. The author calls them basic, I think they are pretty amazing. The next section talks about project level things like configuration, code coverage, file organization and CI integration. The last section went a little over my head and it talked about Plugins, both existing ones and creating your own and then some more advanced parameterization.
Overall the book was very detailed. It was well-organized - one topic lead into the next pretty well. It gave me lots of ideas for what a unit testing solution could be - lots of ideas for features that could be implemented in LUnit or Caraya for example.
Using PyTest with LabVIEW
This may seem odd, but I've actually been using Pytest to test some LabVIEW code lately. The best use case is integration testing for G-CLI Tools. G-CLI tools are meant to be called via the CLI. I can write integration tests for that in LabVIEW. I can use SystemExec to run G-CLI from the Command Line and that works. I get the results back and I can either write some assertions or use approval testing to verify the results. I can easily run those tests from within my project using LUnit or Caraya. The problem comes when I want to use G-CLI to run those same tests from the CLI as part of my CI build process. Recursively calling G-CLI caused to things to hang, so I needed a different solution. I could have just used LabVIEW-CLI to run those tests and that would require writing a special wrapper VI and that seemed too much hassle.
Pytest worked wonderfully for this use case. I just created a fixture to setup the CLI call, then each test used the fixture with the parameters that needed to be passed to the CLI tool for that test. Then I just used approval testing to approve the results. It works very well. If you want to see this in action, you can check out the blue repository.