Python node basics
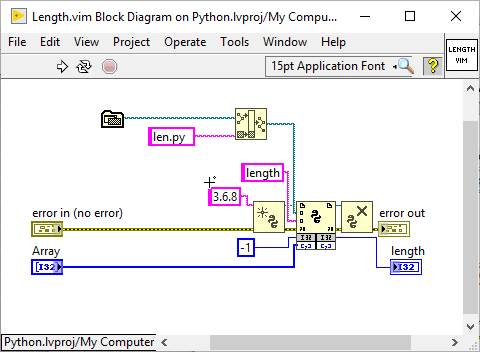
I have been learning a bit about Python lately. I find it a nice complement to LabVIEW. Like LabVIEW it is very good for writing quick little scripts and rapidly prototyping things. Unlike LabVIEW, it is not strictly typed, which makes it rather interesting to me. A few years ago LabVIEW added a Python Node. I thought I would just throw together a few pieces of code to just demonstrate how it works.
The Railroad Tracks
The Python API in LabVIEW looks a lot like most other “railroad track” APIs in LabVIEW. You open Python session by supplying the Python version (note it only supports 2.7 and 3.6.8). That generates a refnum, which you can pass to call the Python node and then close.
NOTE: When installing Python, make sure you select the checkbox to add it to the path.
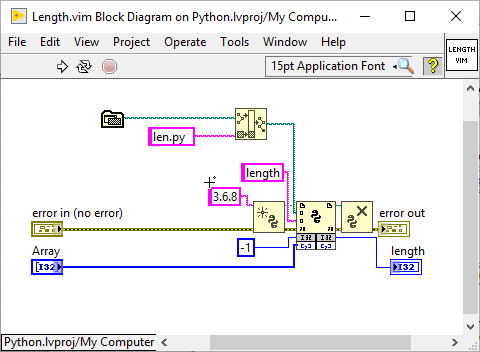
def length(a):
return len(a)
Python Node
The Python Node has several inputs. Of course you have to input a Python session. Then you also have to point it at the Python .py file you want to load and the specific function within that file that you want to run. That is just to set up the function call itself.
You also have to define the output datatype (which is the type of the return value). Then you have to expand it and wire up all of your inputs. Due to Python’s duck typing, the inputs (and the return type) will adapt to whatever you wire to them. If something goes wrong, Python will raise an exception which will get turned into a LabVIEW Error cluster if unhandled inside Python. You can expand the node to add more inputs.
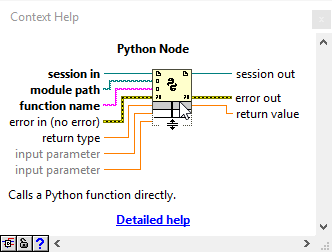
Playing around with VIMs
One of the cool things about the Python node is that since it adapts to type, if you call it inside a VIM then you can pass in virtually anything. (You may want to do some type checking here, depending on your use case.) I put together 2 examples. The first one just calls the len function to return the length of a list or string. So you can pass it a 1-D array (of any datatype) or a string and it will return the length. The next example is the plus operator. If you use the plus operator on 2 numbers, it will add them. If you pass it 2 strings it will concatenate them.
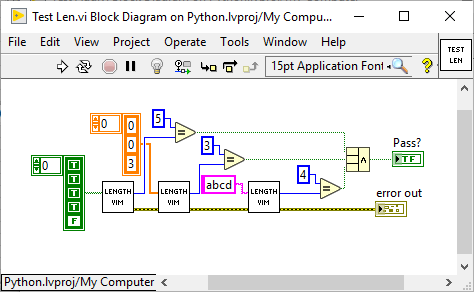
def add(a,b):
return a+b
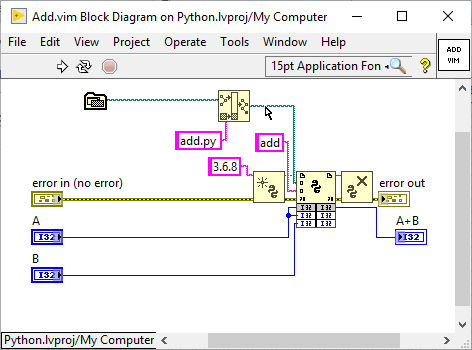
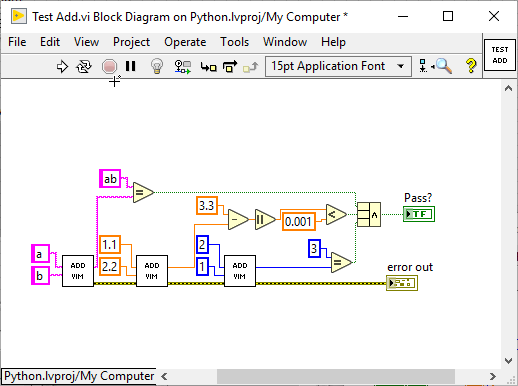
Example code requires LV2020 and Python 3.6.8
If you would like some help integrating Python code into LabVIEW, let’s talk.