OOP Design Patterns in Actor Framework Part 2
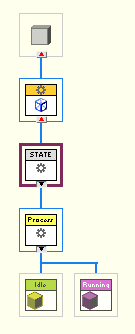
This is a continuation of my previous post on GOF Design Patterns in the Actor Framework. I found a lot of examples, so I decided to break it into 2 posts. You can access part 1 here and my review of the GOF Book here. Part 1 showed examples found in the Actor Framework itself. In this
Proxy
If you have taken the AF class, you may remember that there was a lesson on calling actors from synchronous code. The basic idea was to start with an actor that was written to be called by another actor, so any data it returns is sent via abstract messages. The problem presented is how to reuse this actor in a non-AF synchronous project. The solution was to write a proxy actor that launches your original actor and forwards messages to it. You then hide that actor inside a synchronous API class which is what your synchronous code calls.
When you initialize the synchronous API class, it creates some references (queues, notifiers, etc) and passes them to the proxy actor before launching it and grabbing
Remote Proxy

Network Endpoints are a great example of a remote proxy. The calling actor interacts with the n
State Pattern
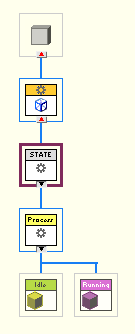
The State Pattern Actor is a great example of an implementation of the state pattern using Actor Framework. The UML above is from the State Pattern Actor Sample Project. The Process Actor represents a State Pattern Actor for a particular process. In this case, the process has 2 states: Idle and Running. Each is a child class of Process Actor. Each can have its own data, handle messages in its own way, and execute logic on
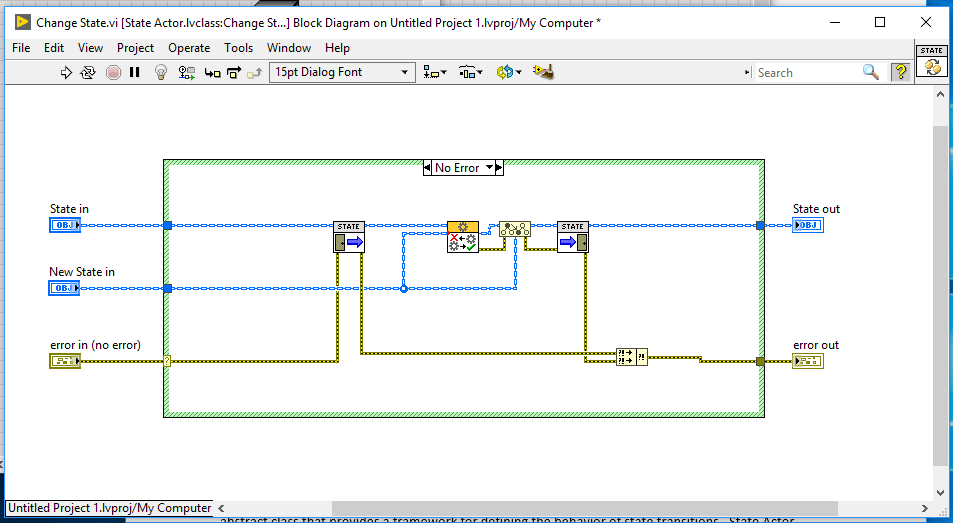
The State Pattern Actor is also a great example of how different patterns can work together. In the UML class diagram above, the State Pattern Actor acts as a template. It makes sure that when changing from one state to another that:
- The current state has the opportunity to execute an action on exit.
- Any data is preserved.
- The new state has the opportunity to execute an action
on enter .
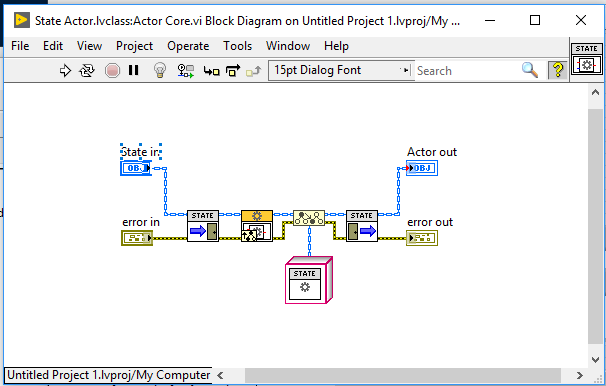
The State Pattern actor core also acts as a template. It makes sure that
Mediator
Ethan Stern has come up with something called the MVA Framework. It is an implementation of the Model View Controller using a variety of GOF patterns, most notably the Mediator pattern. It is built on top of the Actor Framework. The top-level actor is a Controller (which he calls a
Further Reading
Stephen Loftus-Mercer and Eli Kerry have posted LabVIEW examples (non-AF based) of several of the GOF patterns to the NI Forums. You can find them here: